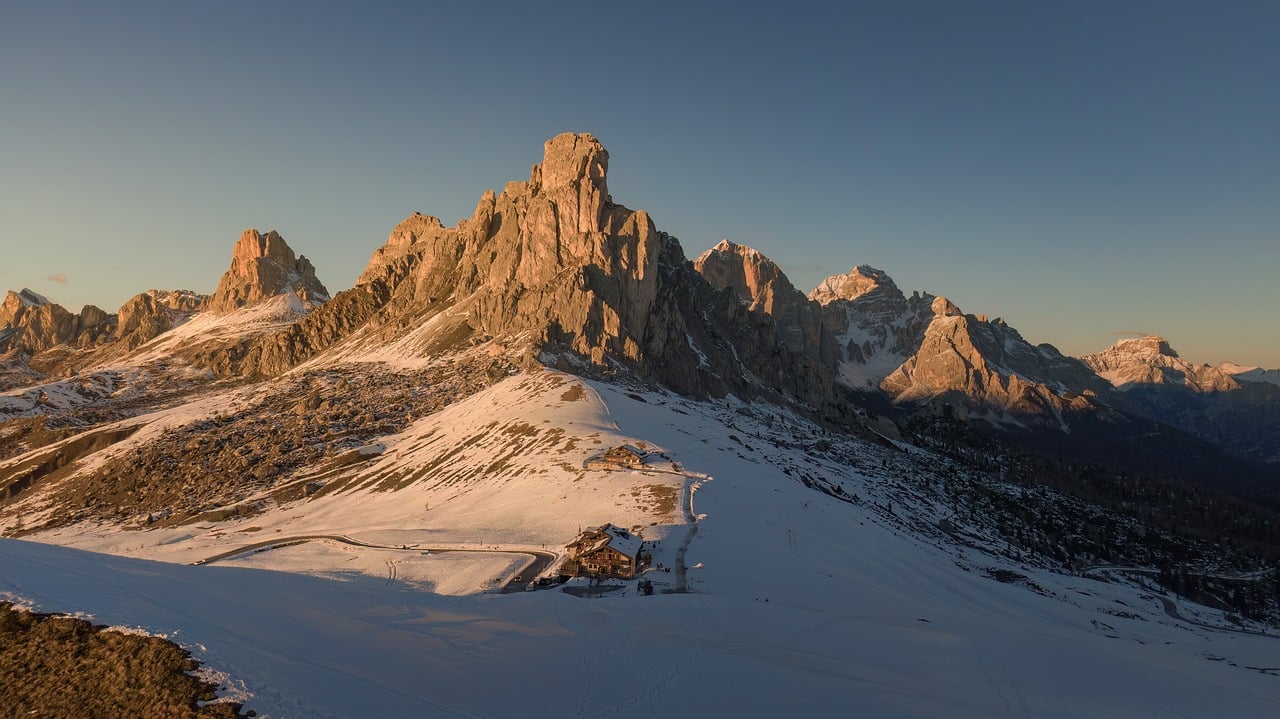
Sun Mar 16 2025 Featured
1487 字 · 9 分钟
Transformers.js 使用经验指南
Table of Contents
介绍 Link to 介绍
Transformers.js 是 Hugging Face 推出的前端机器学习框架,允许开发者直接在浏览器或 Node.js 环境中运行预训练的 Transformer 模型(如 BERT、GPT、Whisper 等)。其核心优势包括:
- 无需后端依赖:直接在前端完成模型推理。
- 跨平台支持:兼容现代浏览器(Chrome、Firefox、Edge)和 Node.js。
- 高性能加速:利用 WebAssembly 和 WebGPU 实现硬件级加速。
- 丰富任务支持:文本分类、语音识别、图像生成等。
安装与引入 Link to 安装与引入
1. 通过 npm 安装 Link to 1. 通过 npm 安装
BASH
1
npm install @xenova/transformers
2. 通过 CDN 引入(无需构建工具) Link to 2. 通过 CDN 引入(无需构建工具)
HTML
123
<script type="module">
import { pipeline } from 'https://cdn.jsdelivr.net/npm/@xenova/transformers@3.1.0';
</script>
核心示例:使用 Pipeline Link to 核心示例:使用 Pipeline
Transformers.js 通过 pipeline 方法快速调用模型。以下为常见任务示例:
示例 1:自动语音识别(ASR) Link to 示例 1:自动语音识别(ASR)
JAVASCRIPT
12345678910
// 加载 Whisper 模型进行语音转文字
const transcriber = await pipeline(
'automatic-speech-recognition',
'Xenova/whisper-tiny'
);
// 处理音频文件(如 happy.mp3)
const audio = 'happy.mp3';
const result = await transcriber(audio);
console.log(result.text); // 输出转录文本
示例 2:文本摘要生成 Link to 示例 2:文本摘要生成
JAVASCRIPT
123456789101112
// 加载文本摘要模型
const summarizer = await pipeline(
'summarization',
'Xenova/distilbart-cnn-12-6'
);
// 生成摘要
const summary = await summarizer(
"输入文本...",
{ min_length: 30, max_length: 150 }
);
console.log(summary[0].summary_text);
示例 3:文本转语音(TTS) Link to 示例 3:文本转语音(TTS)
JAVASCRIPT
123456789101112
// 加载 SpeechT5 模型
const tts = await pipeline(
'text-to-speech',
'Xenova/speecht5_tts',
{ quantized: false } // 禁用量化以提升音质
);
// 生成语音
const audio = await tts("你好,Transformers.js!");
const audioBlob = new Blob([audio.audio], { type: 'audio/wav' });
const audioUrl = URL.createObjectURL(audioBlob);
document.getElementById('audioPlayer').src = audioUrl;
支持的任务类型 Link to 支持的任务类型
任务类型 | 示例模型 | 代码片段 |
---|---|---|
文本分类 | Xenova/distilbert-base-uncased | pipeline('text-classification', ...) |
图像分类 | Xenova/vit-base-patch16-224 | pipeline('image-classification', ...) |
图像生成 | Xenova/stable-diffusion-2-1 | pipeline('text-to-image', ...) |
多模态问答 | Xenova/vilt | pipeline('vqa', ...) |
错误处理与调试 Link to 错误处理与调试
1. 模型加载失败 Link to 1. 模型加载失败
JAVASCRIPT
12345
try {
const pipeline = await pipeline('task', 'model-id');
} catch (error) {
console.error("模型加载失败:", error.message);
}
2. 输入格式错误 Link to 2. 输入格式错误
JAVASCRIPT
1234567
// 检查音频文件格式是否支持
async function transcribeAudio(audioFile) {
if (!audioFile.type.startsWith('audio/')) {
throw new Error("无效的音频文件类型");
}
// 继续处理...
}
性能优化技巧 Link to 性能优化技巧
1. 使用 WebGPU 加速 Link to 1. 使用 WebGPU 加速
JAVASCRIPT
123
// 在支持 WebGPU 的浏览器中自动启用加速
const pipelineOptions = { device: 'webgpu' };
const model = await pipeline('task', 'model-id', pipelineOptions);
2. 模型量化(压缩) Link to 2. 模型量化(压缩)
JAVASCRIPT
123456
// 加载量化后的模型(减少内存占用)
const quantizedModel = await pipeline(
'text-classification',
'Xenova/distilbert-base-uncased',
{ quantized: true }
);
3. 缓存模型 Link to 3. 缓存模型
JAVASCRIPT
123456789
// 避免重复加载模型
let cachedPipeline = null;
async function getPipeline() {
if (!cachedPipeline) {
cachedPipeline = await pipeline('task', 'model-id');
}
return cachedPipeline;
}
最佳实践 Link to 最佳实践
- 选择合适模型:根据任务需求选择轻量级模型(如 tiny 或 base 版本)。
- 处理大模型:对于超大模型(如 Xenova/whisper-large),建议在服务器端预处理。
- 浏览器兼容性:优先使用支持 WebGPU 的浏览器(如 Chrome 100+)。
- 安全与隐私:敏感数据(如音频)应避免直接上传至服务器,优先本地处理。
- 监控资源:使用浏览器开发者工具监控内存和 GPU 使用情况。
总结 Link to 总结
Transformers.js 为前端开发者提供了强大的机器学习能力,但需注意以下几点:
- 模型大小:大型模型可能导致页面卡顿,建议分阶段加载。
- 任务选择:优先使用 Hugging Face Hub 上标注为“Web 推理友好”的模型。
- 社区支持:参考 Transformers.js 官方文档获取最新模型列表和 API 变更。
通过合理使用上述技巧,你可以在浏览器中快速构建智能应用,例如语音助手、实时翻译或图像生成工具。
附录:常见问题 Link to 附录:常见问题
如何查看支持的模型列表?
访问 Hugging Face Hub,筛选“Web 环境支持”的模型。Node.js 中的使用限制?
Node.js 需安装onnxruntime
或tensorflow.js
依赖,且性能可能低于浏览器环境。多任务处理优化?
使用Promise.all
并行执行多个推理任务,例如同时进行文本分类和图像生成。
感谢阅读!